A Quick Approach To Using Sidekiq With Rails on Heroku
Some notes for getting up and running with sidekiq, redis and rails on heroku.
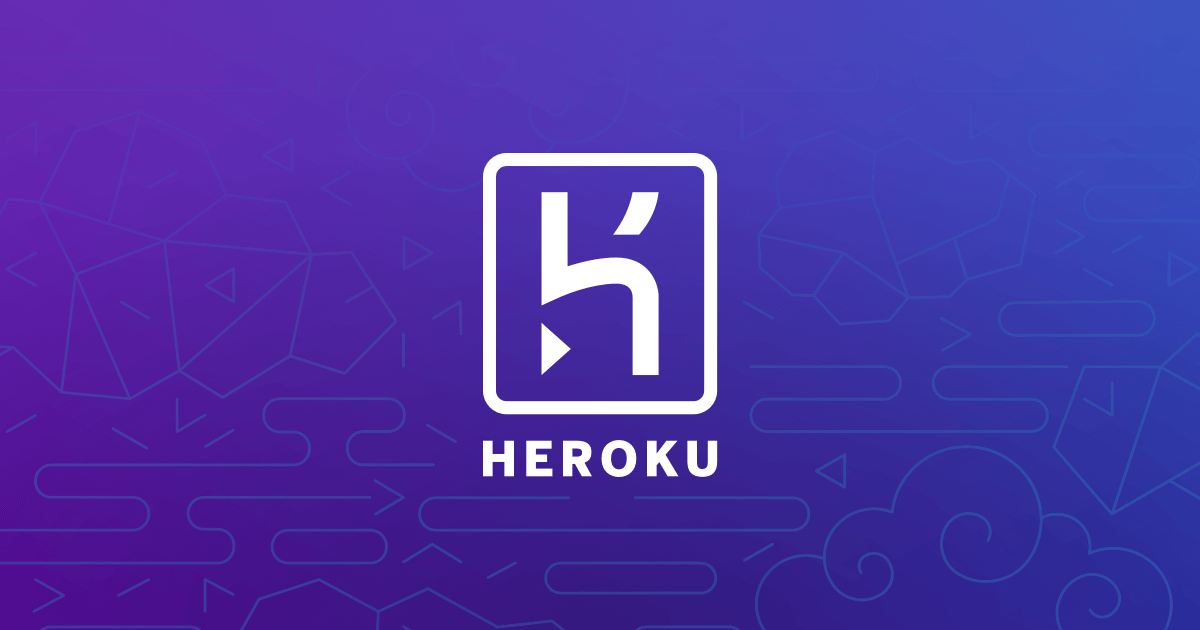
Add the sidekiq gem to your app:
gem 'sidekiq'
bundle install
From your terminal, add the redistogo add-on to your heroku account and set the plan to nano:
heroku addons:create redistogo:nano
Note: At the time of writing this, the current version of redistogo is using redis 3.x but sidekiq 6+ is using redis 4. To get everything to play nicely together, you will unfortunately have to set sidekiq in your app to an earlier version for the time being.
From your terminal, set the REDIS_PROVIDER to equal your REDISTOGO_URL:
heroku config:set REDIS_PROVIDER=REDISTOGO_URL
Create a Procfile and set concurrency to 2 for now so we don't get max connection errors out of the gate:
web: bundle exec rails server -p $PORT
worker: bundle exec sidekiq -c 2 -e production
Before moving on to the next step, you'll need to deploy the current changes we've made to your heroku app.
From your terminal do this:
heroku ps:scale worker=1
Now create a worker:
rails g sidekiq:worker SomeAction
This will generate a new worker in your app. By default your worker will look something like this:
class SomeActionWorker
include Sidekiq::Worker
def perform(args)
# do something!
end
end
Add your code to it, and then you can call your worker where you need to in your code:
SomeActionWorker.perform_async(args)
Deploy that to heroku and try it out! Let me know if it works for you 😉.